I wrote a very tiny MVC Application Framework which runs on OWIN. This Framework uses the Razor engine for the view rendering. It’s very fast because the overhead is quite small. There are just a few lines of code which are between the Owin HTTP server request and the application logic.
For that it doesn’t contain so much functionality as ASP.NET MVC Framework.
This framework handles only HTTP GET requests. HTTP POST is not supported for now.
The code is on GitHub: Razor-enabled MVC Application Framework
The architecture is as follows:
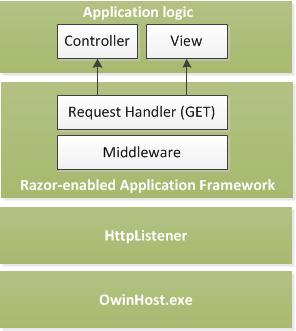
Razor Server – Architecture
The whole thing runs in OwinHost.exe and uses the HttpListener. (Well, it can be exchanged due IIS/Self Host and SystemWeb as server.)
The Razor-enabled MVC Application Framework itself handles the requests and invokes based on the request path (owin.requestPath) the appropriate Controller-Action. The Controller-Action result is passed to the Razor engine. The engine loads the view definition and parses it with data. Afterwards the generated HTML view is written to the response body (owin.ResponseBody). That’s it.
The code below demonstrates how to build an application based on the Razor-enabled MVC Application Framework.
The solution is structured as follows:
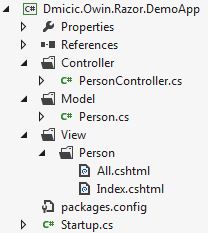
Razor Demo App
First we have to create a Startup class and configure the routes.
public class Startup { public void Configuration(IAppBuilder app) { app.Use(new Func<object, object>( x => new Middleware(new List<Route> { // Your routes new Route("Person", typeof(PersonController)) }) )); } }
Now define your model. In this example: “Person”
public class Person { public string Firstname { get; set; } }
Now define your controller.
public class PersonController { public IView Index() { return new View("Index", new Person() { Firstname = "Max" }); } public IView All() { var result = new List<Person>(); for (var x = 0; x < 150; x++) { result.Add(new Person() { Firstname = "Max " + x }); } return new View("All", result); } }
Almost finished. Now create the views with the Razor syntax.
<html> <head> <title>Razor Server</title> </head> <body> @foreach (var person in Model) { <b>@person.Firstname</b><br /> } </body> </html>
And
<html> <head> <title>Razor Server</title> </head> <body> <b>@Model.Firstname</b> </body> </html>
This is it! Run the application an execute some HTTP requests.
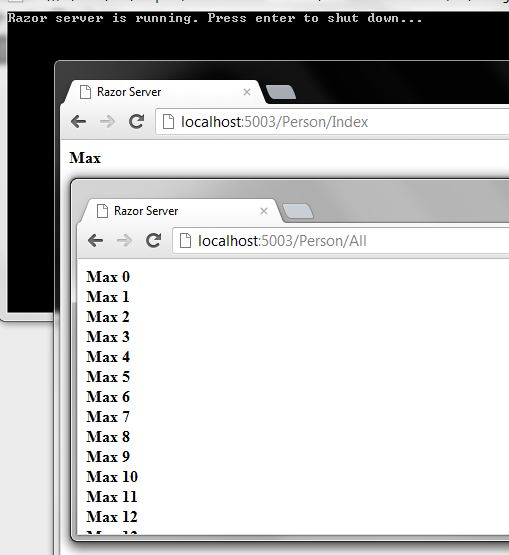
Razor Server HTTP Requests